WebSockets are the backbone of modern realtime applications. But what are WebSockets, how do they work, and are they right for your application at scale?
In this guide we’ll break down what WebSockets are, how they work, test their performance and help you decide if they are right for your realtime architecture. Whether you’re building collaborative tools, live data dashboards or multiplayer games, this page will help you assess WebSockets from both a technical and strategic perspective.
What are WebSockets?
WebSocket is a network protocol that provides full-duplex communication over a single, persistent TCP connection. Unlike HTTP which follows a request-response model, WebSockets enable continuous, low-latency, bi-directional communication between client and server.
This makes them perfect for realtime use cases like:
Live chat applications
Multiplayer gaming
Collaborative document editing
IoT device updates
Financial dashboards
What is the WebSocket API?
The WebSocket API is a browser-based interface that allows web applications to open a persistent connection with a server. It enables the exchange of data in both directions without the need for repeated HTTP requests.
Here is a basic example of using the WebSocket API:
const socket = new WebSocket('wss://example.com/socket');
socket.onopen = function(event) {
console.log('WebSocket connection established');
socket.send('Hello server!');
};
socket.onmessage = function(event) {
console.log('Message from server ', event.data);
};
socket.onclose = function(event) {
console.log('WebSocket connection closed');
};
This API is supported in all modern browsers and is the foundation of many frontend real-time applications.
How do WebSockets work?
WebSocket communication starts with an HTTP handshake. Once the connection is upgraded, it stays open and both the client and server can push data independently at any time.
Step-by-step WebSocket flow:
Client sends an HTTP
Upgrade
request.Server agrees and upgrades to WebSocket protocol.
A persistent connection is established.
Messages can now be sent in either direction with minimal overhead.
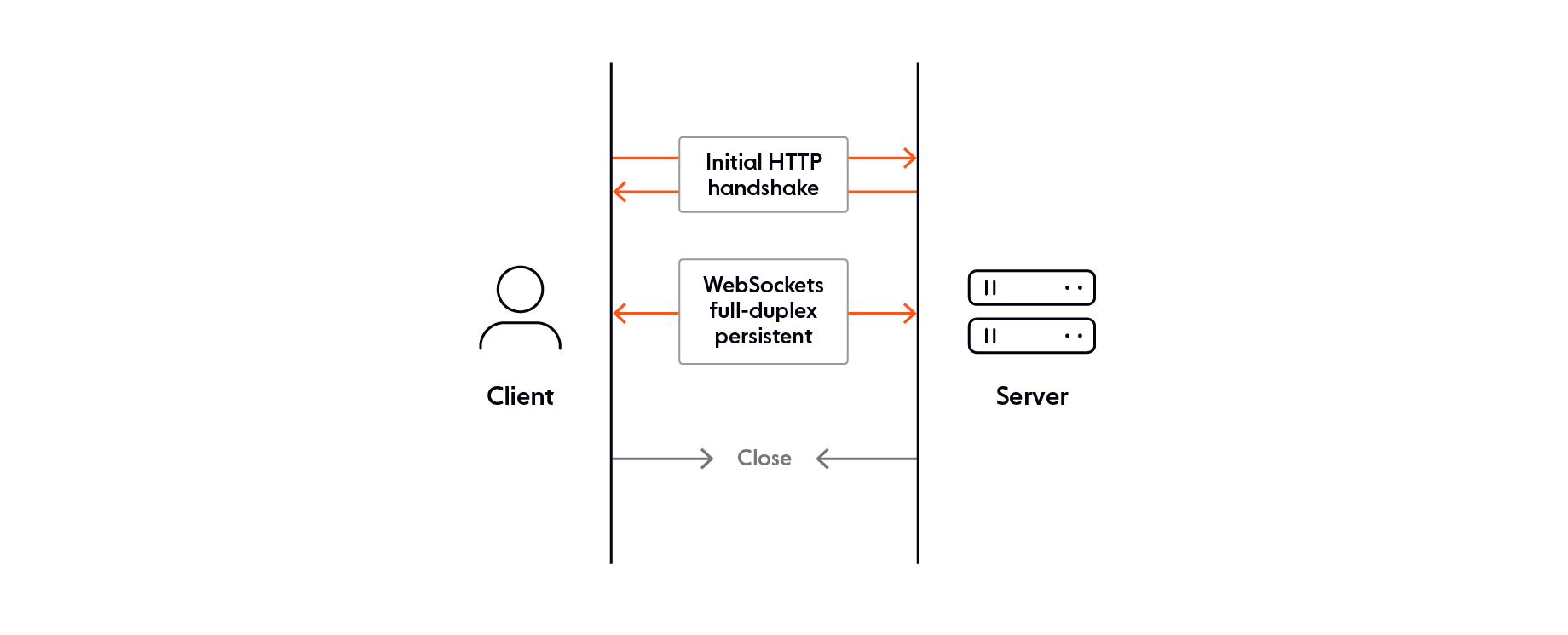
Key WebSocket properties:
Persistent connection (until closed)
Low protocol overhead
Full-duplex communication
Operates over TCP (port 80 or 443)
Getting started with WebSockets
To start building with WebSockets, you can:
1. Use the native WebSocket API
Most modern browsers expose the WebSocket constructor directly. For example:
const ws = new WebSocket('wss://your-app-server.com/socket');
ws.onmessage = (event) => console.log(event.data);
2. Use a managed WebSocket platform
Instead of running your own infrastructure, platforms like Ably provide:
Global edge messaging infrastructure
Client SDKs for JavaScript, iOS, Android, and more
Built-in pub/sub, presence, and history
import * as Ably from 'ably';
const ably = new Ably.Realtime('your-api-key');
const channel = ably.channels.get('chat-room');
channel.subscribe((msg) => console.log(msg.data));
channel.publish('greeting', 'hello');
This approach helps you scale reliably without worrying about reconnection, fallback transports, or data integrity.
Explore: Ably Documentation
WebSocket performance and latency
WebSockets can give you a real performance boost over traditional polling methods. That's because they minimize the overhead of the protocol and keep connections open all the time. But the real-world performance you get depends on a lot of factors, including:
Geographic latency: The physical distance between client and server can impact round-trip time.
Connection scaling: Supporting thousands or millions of concurrent connections requires careful infrastructure planning.
Reliability considerations: Handling network interruptions, ensuring message ordering, and implementing retry mechanisms are essential for production-readiness.
These challenges can be tough to overcome on your own. That's where third-party services come in. They're built to handle WebSocket connections at scale, and can help you sidestep latency, scaling and reliability issues. With a managed solution, you get optimized infrastructure, global distribution and built-in safety nets. That means you can focus on what matters to you.
WebSockets vs alternative protocols
When building a realtime application, WebSockets are often just one of several protocol options available. Each alternative has its own strengths and weaknesses depending on your use case, whether it’s browser compatibility, support for one-way or two-way communication, or handling large-scale IoT environments. The table below compares WebSockets to other popular transport protocols.
Protocol | Use Case Fit | Limitations |
---|---|---|
WebSockets | Realtime messaging, chat | No built-in fallback or ordering |
Server-Sent Events (SSE) | One-way updates | Not bidirectional, no binary support |
gRPC | Microservices, API streaming | Limited browser support |
HTTP/2 | Efficient REST delivery | Still request-response |
MQTT | IoT/embedded devices | Complex broker management |
While the protocols above each serve distinct roles, choosing the right one often comes down to the needs of your architecture, scale, and engineering resources.
Ably supports multiple realtime transport protocols including WebSockets, Server-Sent Events (SSE), and MQTT—allowing you to choose the right technology for your specific use case without managing complex infrastructure.
Considering a third-party WebSocket service? Here's what you need to think about
When evaluating third-party WebSocket services, it's important to go beyond protocol support and assess how well a provider can meet your technical and operational requirements at scale. Here are some key factors you should take into account:
Global infrastructure: Does the provider offer globally distributed edge servers for reduced latency?
Message reliability: Are features like guaranteed ordering, delivery, and retries built-in?
Scalability: Can the platform automatically scale to handle spikes in concurrent connections without degradation?
Protocol and client support: Is there support for WebSockets as well as fallback protocols like SSE or MQTT, along with SDKs for your target platforms?
Security and compliance: Does the platform meet necessary security certifications and compliance standards (e.g., SOC 2, GDPR)?
Monitoring and tooling: Are there dashboards, APIs, and alerts to help you monitor and debug your realtime systems?
Using resources like our comparison pages can help to evaluate the different third-party providers available.
WebSocket FAQs
What is a WebSocket and how does it work?
You can think of a WebSocket connection as a long-lived, bidirectional, full-duplex communication channel between a web client and a web server. Note that WebSocket connections work on top of TCP.
How does a WebSocket differ from HTTP?
HTTP is a stateless request-response model, while WebSocket is a stateful, continuous connection allowing low-latency, two-way communication.
Are WebSockets scalable?
Yes, WebSockets are scalable. Companies like Slack, Netflix, and Uber use WebSockets to power realtime features in their apps for millions of end-users. For example, Slack uses WebSockets for instant messaging between chat users.
However, scaling WebSockets is non-trivial, and involves numerous engineering decisions and technical trade-offs. Among them:
Should you use vertical or horizontal scaling?
How do you deal with unpredictable loads?
How do you manage WebSocket connections at scale?
How much bandwidth is being used overall, and how is it impacting your budget?
Do you have to deal with traffic spikes, and if so, what is the performance impact on the server layer?
How will you automatically add additional server capacity if and when it’s needed?
How do you ensure data integrity (guaranteed message ordering and delivery) at scale?
Are WebSockets secure?
WebSockets can be secure if they are implemented with appropriate security measures. Secure WebSocket connections use the "wss://" URI. This indicates that the connection is encrypted with SSL/TLS, which ensures that the data transmitted between the WebSocket client and WebSocket server is encrypted and cannot be intercepted or tampered with by third parties.
Additionally, WebSocket connections can be subject to the same security policies as HTTP connections, such as cross-origin resource sharing (CORS) restrictions, which prevent unauthorized access to resources across different domains.
Note that the WebSocket protocol doesn’t prescribe any particular way for servers to authenticate clients. For example, you can handle authentication during the opening handshake, by using cookie headers. Another option is to manage authentication (and authorization) at the application level, by using techniques such as JSON Web Tokens.
Learn more about common WebSocket security vulnerabilities - and how to prevent them
Are WebSockets faster than HTTP?
In the context of realtime apps that require frequent data exchanges, WebSockets are faster than HTTP.
HTTP connections have additional overhead, such as headers and other metadata, that can add latency and reduce performance compared to WebSocket connections, which are designed for persistent, low-latency, bidirectional communication. With WebSockets, there’s no need for multiple HTTP requests and responses. This can result in faster communication and lower latency.
Learn more about the differences between WebSockets and HTTP
Are WebSockets synchronous or asynchronous?
WebSockets are asynchronous by design, meaning that data can be sent and received at any time, without blocking or waiting for a response. However, it's important to note that while WebSockets themselves are asynchronous, the code used to handle WebSocket events and messages may be synchronous or asynchronous, depending on how it’s written.
Are WebSockets expensive?
A WebSocket connection is not inherently expensive, as it's designed to be lightweight and efficient, with minimal overhead. That being said, building and managing a scalable and reliable WebSocket system in-house is expensive, time-consuming, and requires significant engineering effort:
10.2 person-months is the average time to build basic WebSocket infrastructure, with limited scalability, in-house.
Half of all self-built WebSocket solutions require $100K-$200K a year in upkeep.
Learn more about what it costs to build WebSocket infrastructure in-house
Ready to build realtime at scale?
Recommended Articles

The challenge of scaling WebSockets [with video]
Learn how to scale WebSockets effectively. Explore architecture, load balancing, sticky sessions, backpressure, and best practices for realtime systems.
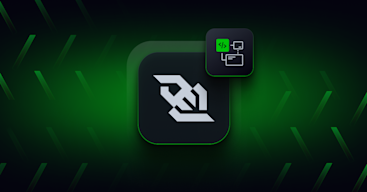
WebSocket architecture best practices: Designing scalable realtime systems
Learn WebSocket architecture best practices to design robust realtime systems. Improve scalability and performance with proven strategies.
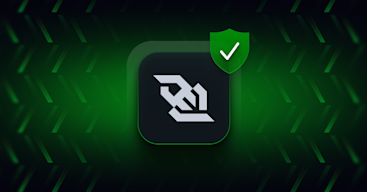
WebSocket reliability in realtime infrastructure
How reliable are WebSockets, and what does it take to build a reliable WebSocket infrastructure? We'll explore this and the considerations to make on whether to use a PaaS.